# Array - reverse
Welcome back to our Web Basics, a series on essential programming topics every web developer should absolutely know!
In today’s lesson, let’s learn about reverse() — This method is used to reverse the order of the elements in an array. And yup, it can be used for array of any types.
const letters = ['a', 'b', 'c'];
letters.reverse();
// ['c', 'b', 'a'];
# Examples
# Array of Numbers
const nums = [2, 10, 5];
nums.reverse;
// [5, 10, 2]
# Array of Emojis
Nope, "emojis" aren't a type. It's just a string. Notice the quotation around it. It's important to have fun in programming, I find emojis always help 😝
const medals = ['🥇', '🥈', '🥉'];
medals.reverse();
// ['🥉' , '🥈', '🥇' ]
# Array of Mixed Types
const mix = [true, 'hi', null, 100, undefined];
mix.reverse();
// [ undefined, 100, null, 'hi', true ]
# Resources
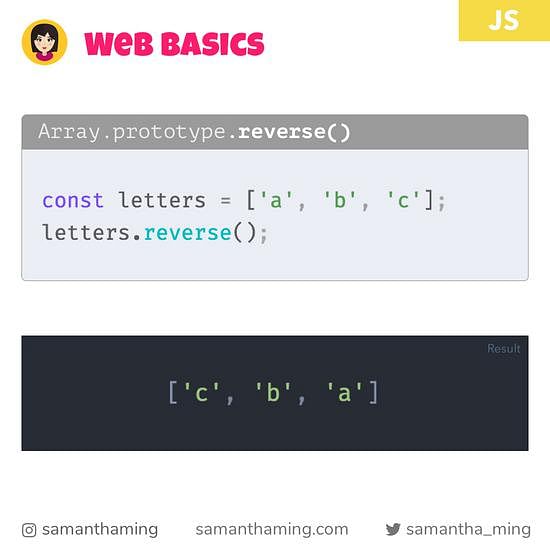